TLDR; ‘Docker demystified’ is for newbies who want to dive into the world of containerization with Docker. I’ll explain the concept of containers, how Docker simplifies deployment, and walk through setting up your first Docker container. I’ll wrap with a practical demonstration of automating a simple app deployment.
Understanding containerization
In the ever-evolving world of software development, the need for efficient, scalable, and consistent deployment methods is key. This is where Docker, a powerful containerization platform, comes in. But before diving into Docker, it’s important to understand the concept of containerization. Containerization is a method of packaging software so that it can run consistently on any environment. It isolates applications and their dependencies into a self-contained unit called a container. This approach ensures that the software works uniformly despite differences in OS and underlying infrastructure.
Why use Docker?
Docker simplifies the process of creating, deploying, and running applications using containers. Here’s why that matters:
- Consistency: Ensures applications run the same in different environments.
- Scalability: Easily scale up or down as per demand.
- Isolation: Each container operates independently, reducing conflicts.
- Efficiency: Utilizes system resources more effectively than traditional virtualization.
Setting up Docker
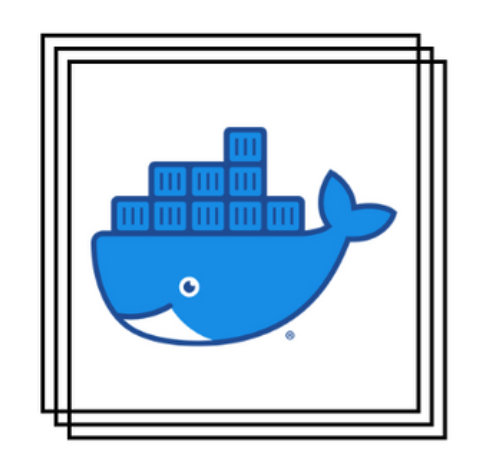
To get started with Docker, you need to install Docker Desktop on your machine. It’s available for Windows, Mac, and Linux. Download it from the official Docker website.
Your first Docker container
Let’s create a basic Node.js application and containerize it.
Step 1: Create a Simple Node.js Application
Create a new directory and initialize a Node.js application:
mkdir my-node-app
cd my-node-app
npm init -y
Create an index.js
file with the following content:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello from Docker!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Step 2: Dockerize the app
Create a Dockerfile
in the root of your project:
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "index.js"]
This Dockerfile
does the following:
- Base Image: Starts from a Node.js image (
node:14
). - Working Directory: Sets the working directory in the container.
- Copy Files: Copies package files and then all other files.
- Install Dependencies: Installs Node.js dependencies.
- Expose Port: Exposes port 3000.
- Start Command: Specifies the command to run the application.
Step 3: Build and run the Docker container
Build the Docker image:
docker build -t my-node-app .
Run the container:
docker run -p 3000:3000 my-node-app
Visit http://localhost:3000
in your browser. You should see “Hello from Docker!”
Automating with Docker Compose
For more complex applications, Docker Compose can automate the deployment of multiple containers. Let’s create a docker-compose.yml
file:
version: '3'
services:
web:
build: .
ports:
- "3000:3000"
Run the application using Docker Compose:
docker-compose up
Docker offers a streamlined, efficient way to containerize apps, ensuring they run consistently across environments. You should now be able to set up a basic Docker container and automate deployments using Docker Compose. In a future post we will dive deeper and talk about networking, data persistence, and optimizing Dockerfiles for production. Happy containerizing!