TLDR; Javascript is the backbone of web development. It’s ubiquitous and flexible and easy to learn, but its flexibility is a double-edged sword. Just ask anyone who has tried to scale a moderately complex app. Typescript is like the grownup version of Javascript. It has more structure and is designed to ensure type safety and reliability. In this post I’ll dive into why Typescript was conceived, its evolution, and how it enhances Javascript projects.
The Pitfalls of Pure Javascript
Imagine you’re building a web app. You have multiple devs working on the project and the codebase is growing. You’ve just written a function to fetch user data and it looks like this in Javascript:
function getUser(id) {
// Fetch user data from an API
return fetch(`api/users/${id}`).then(response => response.json());
}
const user = getUser(1);
console.log(user.name); // What if user is undefined or doesn’t have a name property?
This code is straightforward, but you can see the potential issues. What if the user
is undefined
or doesn’t have a name
property? Javascript won’t catch these errors until runtime, which can lead to more bug bashing than you should ever have to do in production.
Enter Typescript 💪
Typescript was developed by Microsoft, first released in 2012, to address such issues. It was designed to bring static type-checking to Javascript, allowing developers to catch errors early during the development phase. Typescript is a superset of Javascript, which is a fancy way of saying that all Javascript code is valid Typescript, but Typescript adds additional features, primarily around type safety.
Types of Types! Understanding Typescript’s Type System
I worked on a project a while back with a dev who was using Typescript for the first time. He sprinkled any
all over the code like fairy dust and I was like, bro… No.
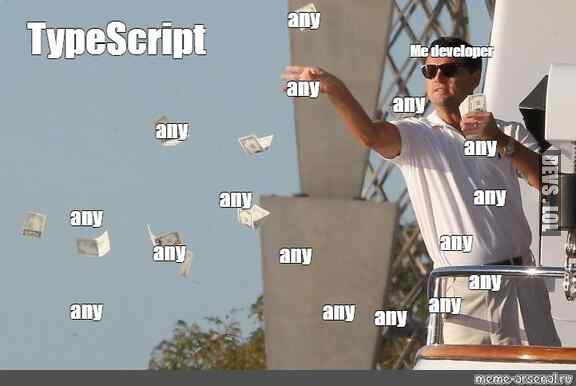
TypeScript introduces several types that help in making the code more robust and self-documenting (my favorite aspect). Some common types include:
- Basic Types: Number, String, Boolean, Void, Null, Undefined
- Object Types: Arrays, Functions, Classes
- Special Types: Any, Unknown, Never, Enum, Tuple
These types help define the shape and expected values of your variables, parameters, and return values. NOTE: it’s very important to understand the distinction between primitive and non-primitive types for writing effective code. This goes to how these types are stored and manipulated.
Primitive Types
Primitive types are the most basic data types. They are immutable and passed by value. This means when you manipulate a primitive type, you work directly with its value. Typescript’s primitive types include:
- Number: Represents numeric values (e.g.
let age: number = 30
) - String: Represents textual data (e.g.
let name: string = 'Alice'
) - Boolean: Represents true/false values (e.g.
let isActive: boolean = true
) - Null: Represents the intentional absence of any object value (e.g.
let empty: null = null
) - Undefined: Indicates variables that have not been initialized (e.g.
let notDefined: undefined
) - Symbol (introduced in ES6): Represents unique, immutable identifiers (e.g.
let sym: symbol = Symbol('key')
)
Non-Primitive Types
Non-primitive types, also known as reference types, include objects, arrays, functions, and classes. Unlike primitives, non-primitives are mutable and are passed by reference. This means when you manipulate a non-primitive type, you work with a reference to its memory location, not the actual value!
- Object: The most generic type for any Javascript object (e.g.
let user: object = {name: 'Alice', age: 30}
) - Array: Represents a collection of values (e.g.
let numbers: number[] = [1, 2, 3]
) - Function: A callable set of statements (e.g.
let greet: (name: string) => string
) - Class: A blueprint for creating objects (e.g.
class User {...}
)
Avoiding Type Misuse
Understanding types of types helps avoid using the wrong types in your code. Also, do yourself a favor and Avoid any
: The any
type bypasses Typescript’s type checking, so really what is the point. Here’s an example of the correct usage of primitive and non-primitive types:
// Correct usage of primitive type
let age: number = 30;
// Incorrect: assigning a string to a number variable
// age = "thirty"; // TypeScript will flag this as an error
// Correct usage of non-primitive type
let user: { name: string; age: number } = { name: 'Alice', age: 30 };
// Using type guards
if (typeof age === "number") {
console.log("Age is a number.");
}
Types vs. Interfaces
In Typescript, both types and interfaces are used to define the shape of an object or a function. However, they have different use cases:
- Types are typically used for unions, intersections, and to define the shape of data.
- Interfaces are better for declaring the shape of objects and are more extensible.
Here’s a type example:
type User = {
id: number;
name: string;
};
const user: User = {
id: 1,
name: 'Alice'
};
And here’s an example of using an Interface:
interface IUser {
id: number;
name: string;
}
const user: IUser = {
id: 1,
name: 'Bob'
};
I know. They look identical, but remember you would want to use type
to combine different types or to define a type that could be one of several things. Here’s an example:
// Define basic types
type StringOrNumber = string | number;
type UserStatus = 'active' | 'inactive' | 'suspended';
// Define a more complex type combining object and union types
type User = {
id: StringOrNumber;
name: string;
status: UserStatus;
};
// Example usage
const user1: User = {
id: 123,
name: 'Alice',
status: 'active'
};
const user2: User = {
id: '124AB',
name: 'Bob',
status: 'suspended'
};
// Function that takes StringOrNumber type
function printId(id: StringOrNumber) {
console.log(`User ID: ${id}`);
}
printId(user1.id); // Outputs: User ID: 123
printId(user2.id); // Outputs: User ID: 124AB
On the other hand, interface
is ideal for declaring the shape of objects, particularly when you expect to extend or implement them in classes. Interfaces are better suited for object-oriented programming because they can be extended or implemented, allowing for more structured and hierarchical type definitions. Additionally, interfaces can be augmented by declaring the same interface multiple times, which is particularly useful when working with third-party type declarations or extending existing declarations.
Adding Type Safety to Your Project
Adding Typescript to an existing Javascript project involves a few steps:
- Install Typescript
npm install typescript --save-dev
2. Create a tsconfig.json File
This file configures the Typescript compiler (lots of options here, I will try to cover this in a separate post). You can generate a default one like this:
npx tsc --init
3. Start Converting Files
Rename your .js
files to .ts
(or .jsx
to .tsx
in the case of React), then add types to your variables and functions.
4. Compile Typescript to Javascript
Run the TypeScript compiler to convert .ts
files to .js
.
npx tsc
In sum, Typescript makes Javascript more lovable by adding type safety, which leads to more reliable and maintainable code which leads to happy and sane devs. It’s a powerful tool especially when working on large projects. Typescript requires a bit of a learning curve, but the benefits should become obvious to those who make the leap. Personally I use Typescript in all my projects and try to keep things consistent without getting overly philosophical about it. Like any tool, best to use it for its intended purpose. Happy Typing!